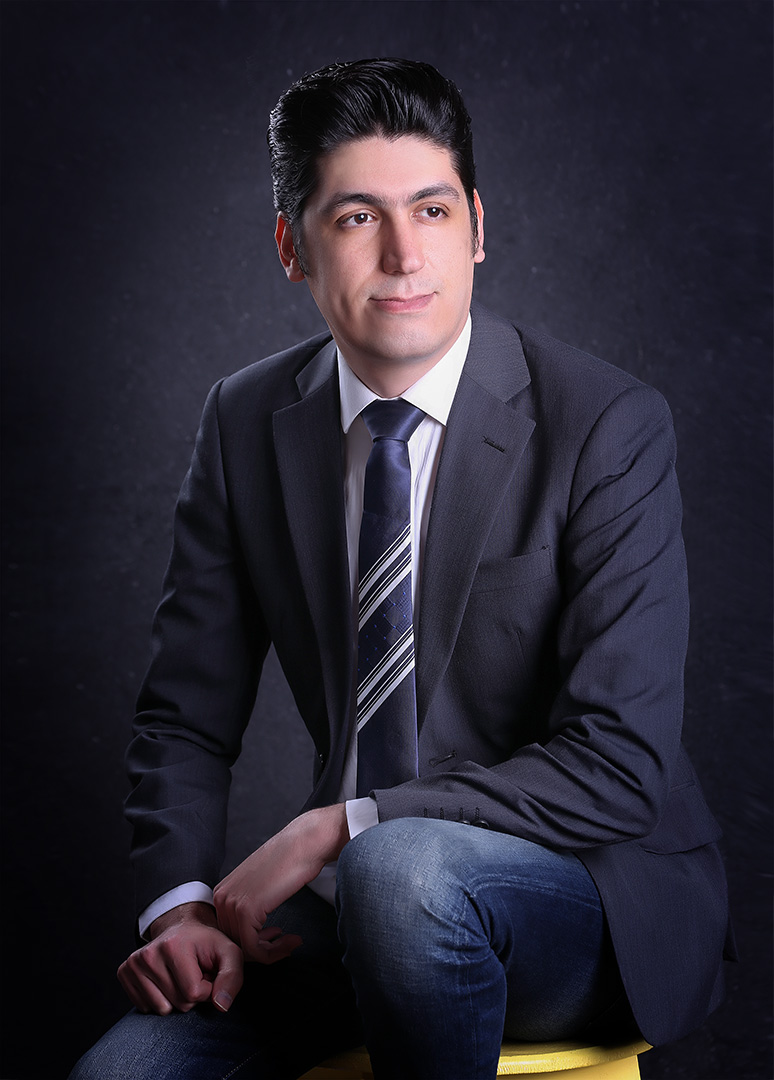
I love programming, football, music and movies. Mostly a full stack web developer, Real Madrid and RHCP fan among other things.
I love programming, football, music and movies. Mostly a full stack web developer, Real Madrid and RHCP fan among other things.
Author: Alireza Noori
Suppose in your Razor Page you need the client to send arbitrary number of files in a single post request.
If you have the following model:
public class ProjectCreateViewModel
{
public string[] Names { get; set; }
public IFormFile[] Images { get; set; }
}
Unfortunately the Asp.Net Core for some reason can fill the Names field but not the Images field. The trick to this, is to bind to a list in the Page's model, and copy the result to the ViewModel. Like this:
public class CreateModel : PageModel
{
[BindProperty]
public ProjectCreateViewModel Input { get; set; }
[BindProperty]
public List ImageList { get; set; }
}
public async Task OnPostAsync()
{
if (ModelState.IsValid)
{
Input.Images = ImageList?.ToArray();
var result = await service.CreateAsync(Input);
if (result)
{
return RedirectToPage("./Index");
}
}
return Page();
}
//on the page:
<input type="file" asp-for="ImageList">
Hope this helps.